SOAP Lua Agent
Introduction
The SoapLuaAgent is an asynchronous helper for Lua scripts running within the LogicApp. It is used for sending out SOAP client requests.
- The SOAP Agent may be used by any Lua script, regardless of which Service initiated that script.
- For receiving SOAP server requests you need to use the SoapLuaService Service.
The SoapLuaAgent communicates with one or more instances of the SoapClientApp which can be used to communicate with one or more external SOAP servers.
The SoapLuaAgent communicates with the SoapClientApp using the SOAP-C-… messages.
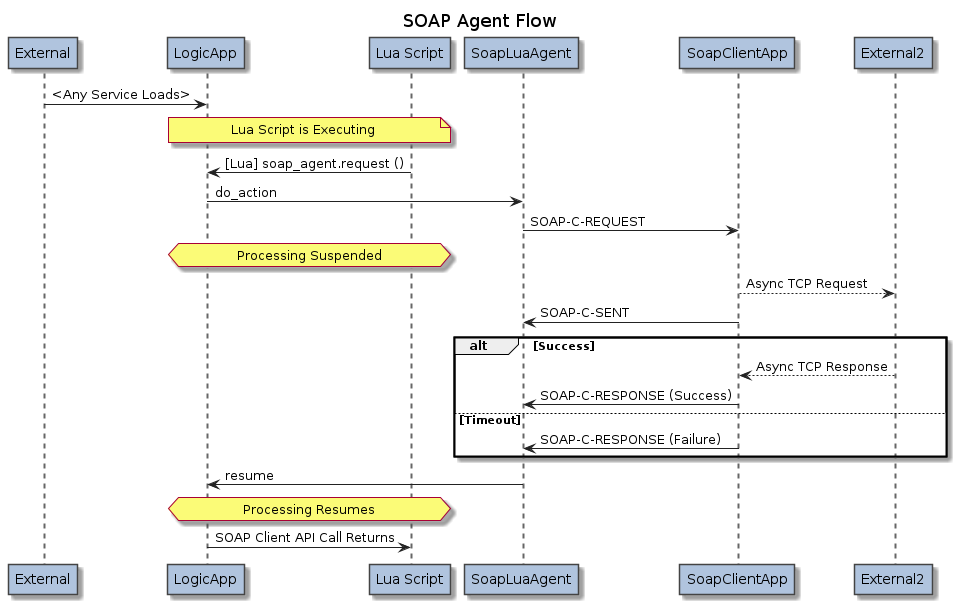
SOAP Agent API methods are accessed via the “n2.n2svcd.soap_agent” module:
local soap_agent = require "n2.n2svcd.soap_agent"
Configuring SoapLuaAgent
The SoapLuaAgent is configured within a LogicApp.
<?xml version="1.0" encoding="utf-8"?>
<n2svcd>
...
<applications>
...
<application name="Logic" module="LogicApp">
<include>
<lib>../apps/logic/lib</lib>
</include>
<parameters>
...
<parameter name="default_soap_app_name" value="SOAP-CLIENT"/>
</parameters>
<config>
<services>
...
</services>
<agents>
<agent module="SoapClientApp::SoapLuaAgent" libs="../apps/soap/lib"/>
</agents>
</config>
</application>
...
</application>
...
</n2svcd>
Under normal installation, following agent
attributes apply:
Parameter Name | Type | XML Type | Description |
---|---|---|---|
module
|
SoapClientApp::
SoapLuaAgent
|
Attribute | [Required] The module name containing the Lua Agent code. |
libs
|
../apps/soap/lib
|
Element |
Location of the module for SoapLuaAgent.
|
In addition, the SoapLuaAgent must be configured with the name of the SoapClientApp with which
it will communicate. This is configured within the parameters
of the containing
LogicApp
.
Parameter Name | Type | XML Type | Description |
---|---|---|---|
parameters
|
Array | Element |
Array of name = value Parameters for this Application instance.
|
.default_soap_app_name
|
String | Attribute | Default name for the SoapClientApp with which SoapLuaAgent will communicate. |
.soap_app_name_
|
String | Attribute |
Use this format when SoapLuaAgent will communicate with more than one SoapClientApp .(Default = SoapLuaAgent uses only the default route/SOAP end-point). |
The SoapLuaAgent API
All methods may raise a Lua Error in the case of exception, including:
- Invalid input parameters supplied by Lua script.
- Unexpected results structure returned from SoapClientApp.
- Processing error occurred at SoapClientApp.
- Timeout occurred at SoapClientApp.
.request [Asynchronous]
The request
method accepts an request
object parameter with the following attributes.
Field | Type | Description |
---|---|---|
request
|
Object | Container for the parameters of the SOAP request that we are to send. |
.path
|
String |
The URI path with which the request should be sent. (Default = Path as configured in the SoapClientApp) |
.query
|
String |
The URI query string (the part following "?" in the URI). (Default = None) |
.fragment
|
String |
The URI fragment string (the part following "#" in the URI). (Default = None) |
.action
|
String | [Required] The SOAPAction header value. |
.message_name
|
String | [Required] The SOAP Request message name. |
.args
|
Object |
Table of message arguments to encode. (Default = No message arguments) |
.http_headers
|
Array of Objects
|
Additional user-level header objects to apply to an outbound HTTP Request (see below for object structure). These headers are added after any headers defined by static RestClientApp application configuration.You should not set the Content-Type or Content-Length headers.(Default = No Additional Headers) |
Each object in the request.http_headers
Array has the following structure.
Field | Type | Description |
---|---|---|
.name
|
String
|
[Required] The name of the HTTP Request header. |
.value
|
String
|
[Required] The full string value of the HTTP Request header. |
.replace
|
0 / 1
|
If 1 then all previous headers of this name are removed, and this header replaces them.(Default = Append to the existing headers, do not replace) |
The request
method returns an response
object with the following attributes.
Parameter | Type | Description |
---|---|---|
response
|
Object |
Container for response fields copied from the SOAP-C-RESPONSE message.
|
.result_name
|
String | The name of the returned result object. |
.args
|
Object | Table of received args. |
.result_namespace
|
Object | A container for the result namespace information. |
.uri
|
String | The result namespace URI, if one was returned. |
.location
|
envelope /message
|
Location of the result namespace URI, if one was used. |
.http_headers
|
Array of Object |
The list of HTTP headers parsed from the HTTP Response (see below for object structure). All HTTP headers are present, including Content-Length and Content-Type .If a HTTP header was repeated in the HTTP Response, then it is repeated in this Array. |
Each object in the response.http_headers
Array has the following structure.
Field | Type | Description |
---|---|---|
.name
|
String
|
[Required] The name of the HTTP Response header. |
.value
|
String
|
[Required] The full string value of the HTTP Response header. |
Example (SOAP Client Request):
-- Base and built-in actions.
local json = require "json"
local n2svcd = require "n2.n2svcd"
local soap_agent = require "n2.n2svcd.soap_agent"
local soap = ...
local response = soap_agent.request ({
path = '/Pack/Path',
action = 'http://tcl-sms/wsdls/Boss/OperationSet1.wsdl',
message_name = 'BuyValuePackRequest',
args = {
CC_Calling_Party_Id = "64220635462",
Reason = "My Very Good Reason"
},
})
if (response.result_name ~= 'BuyValuePackResponse') then
error ("Unexpected .")
end
if (not response.args or not response.args.PACK_NAME) then
error ("Response PACK_NAME is missing.")
end
return { SUCCESS = 1 }