RADIUS Lua Agent
Introduction
The RadiusLuaAgent is an asynchronous helper for Lua scripts running within the LogicApp. It is used for sending out RADIUS client requests.
- The RADIUS Agent may be used by any Lua script, regardless of which Service initiated that script.
The RadiusLuaAgent communicates with one or more instances of the RadiusApp which can be used to communicate with one or more external RADIUS servers.
The RadiusLuaAgent communicates with the RadiusApp using the RADIUS-C-… messages.
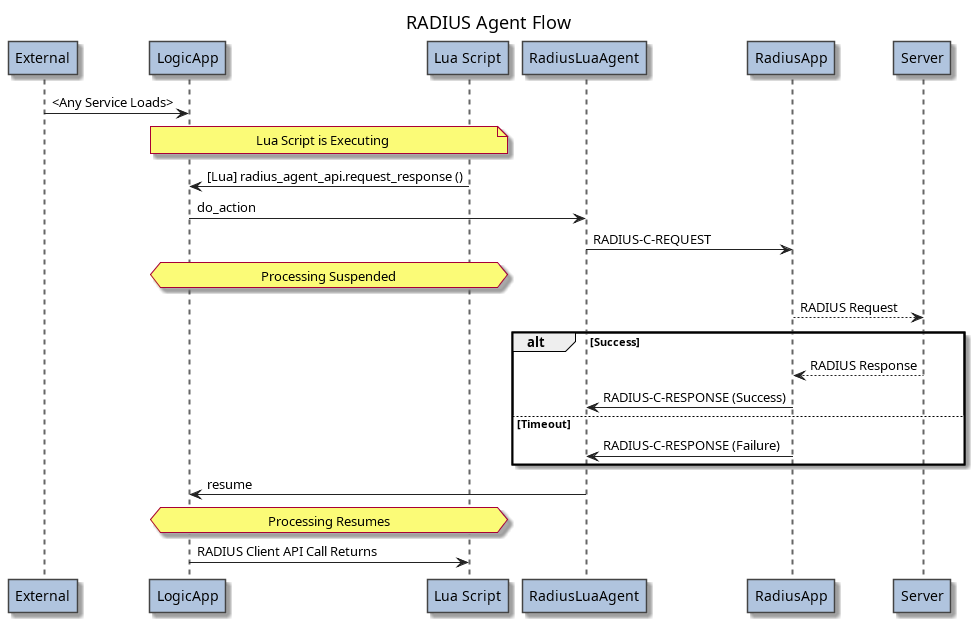
RADIUS Agent API methods are accessed via the “n2.n2svcd.radius_agent” module:
local radius_agent_api = require "n2.n2svcd.radius_agent"
Configuring RadiusLuaAgent
The RadiusLuaAgent is configured within a LogicApp.
<?xml version="1.0" encoding="utf-8"?>
<n2svcd>
...
<applications>
...
<application name="Logic" module="LogicApp">
<include>
<lib>../apps/logic/lib</lib>
</include>
<parameters>
...
<parameter name="default_radius_app_name" value="RADIUS-CLIENT-AUTH"/>
<parameter name="radius_app_name_auth" value="RADIUS-CLIENT-AUTH"/>
<parameter name="radius_app_name_acct" value="RADIUS-CLIENT-ACCT"/>
</parameters>
<config>
<services>
...
</services>
<agents>
<agent module="RadiusApp::RadiusLuaAgent" libs="../apps/radius/lib"/>
</agents>
</config>
</application>
...
</application>
...
</n2svcd>
Under normal installation, following agent
attributes apply:
Parameter Name | Type | XML Type | Description |
---|---|---|---|
module
|
RadiusApp::
RadiusLuaAgent
|
Attribute | [Required] The module name containing the Lua Agent code. |
libs
|
../apps/radius/lib
|
Attribute |
Location of the module for RadiusLuaAgent.
|
In addition, the RadiusLuaAgent must be configured with the name of the RadiusApp with which
it will communicate. This is configured within the parameters
of the containing
LogicApp
.
Parameter Name | Type | XML Type | Description |
---|---|---|---|
parameters
|
Array | Element |
Array of name = value Parameters for this Application instance.
|
.default_radius_app_name
|
String | Attribute | Default name for the RadiusApp with which RadiusLuaAgent will communicate. |
.radius_app_name_<route>
|
String | Attribute |
Use this format when RadiusLuaAgent will communicate with more than one RadiusApp .
|
The RadiusLuaAgent API
All methods may raise a Lua Error in the case of exception, including:
- Invalid input parameters supplied by Lua script.
- Unexpected results structure returned from RadiusApp.
- Processing error occurred at RadiusApp.
- Timeout occurred at RadiusApp.
.request_response [Asynchronous]
The request_response
method sends a RADIUS request, and returns the response. The following parameters are accepted.
Parameter | Type | Description |
---|---|---|
route
|
String |
Optional RADIUS route to use to submit the message. If undefined the default route will be used. (Default = undef )
|
packet_type
|
String or Integer |
[Required] The String packet type name or Integer packet type code of the request to send. If specifying a packet type name, this must be the name of a supported packet type within N2::RADIUS::Codec .
|
attributes
|
Array of Object | Array of RADIUS attribute objects, each with the following fields. |
[].name
|
String |
The name of a RADIUS attribute, e.g. User-Name .This must be the name of a supported attribute within N2::RADIUS::Codec .If the attribute is not supported, you may instead specify [].type and [].data_type .
|
[].type
|
Integer |
The type identifer of the RADIUS attribute, if not specifying by [].name .
|
[].vendor_id
|
Integer |
The identifier of the vendor that defines the attribute if the attribute is a vendor-specific attribute. This field is optional and can be used to disambiguate attribute definitions when [].name and/or [].type are not unique.
|
[].data_type
|
String |
One of enum , ifid , integer , ipv4addr , ipv4prefix ,
ipv6addr , ipv6prefix , string , other , text ,
time .This field is used only when the attribute is not supported in N2::RADIUS::Codec .
For supported attributes the data type is pre-defined.
|
[].value
|
Various |
The value for this attribute. For most attributes this will be a SCALAR of the appropriate type. For attributes with data type other this may be a table.
|
[].value_name
|
String |
This field can be used to specify the value by constant name for attributes with [].data type value enum that have their value mapping defined in N2::RADIUS::Codec .
|
auto_acct_session_id
|
0 /1
|
Flag to indicate if the Acct-Session-Id attribute should be automatically included. (Default = 0 )
|
The request_response
method returns a RADIUS response
object with the following fields.
Field | Type | Description |
---|---|---|
.name
|
String |
The name of the RADIUS packet type, e.g. Access-Accept .This field will only be populated if the packet type is supported within N2::RADIUS::Codec .
|
.code
|
Integer | [Required] The packet type identifier from the received response. |
.identifier
|
Integer | [Required] The identifier field from the received response. |
.length
|
Integer | [Required] The length field from the received response. |
.authenticator
|
String | [Required] The authenticator field from the received response. |
.attributes
|
Array of Object | Array of RADIUS attribute objects, each with the fields described as for a request. |
Example (RADIUS Client Request):
local n2svcd = require "n2.n2svcd"
local radius_agent_api = require "n2.n2svcd.radius_agent"
local soap_args = ...
local result = radius_agent_api.request_response ('auth', 'Access-Request', {
{ name = "User-Name", value = "John.Smith" },
{ name = "User-Password", value = "secret_password" },
{ type = 42, vendor_id = 42, data_type = "integer", value = 789 }
}, false)
soap_response = {}
soap_response['status'] = 'ok'
return soap_response
The above example shows a LogicApp
script invoked by a SoapLuaService.
Note that the decoded .attributes
array will contain all fields, i.e. the [].name
,
[].type
, [].data_type
etc. will all be present.
The only exception will be when decoding attributes which are not part of the core fields
defined within N2::RADIUS::Codec
. In these cases, the [].name
, [].vendor_id
, and [].value_name
fields
will not be present, the [].data_type
field value will be unknown
, and the [].value
will
be the raw undecoded attribute value bytes without any interpretation.
.get_first_attribute [Synchronous]
The get_first_attribute
method searches an array of attributes, returning the first attribute with matching name or type. The following parameters are accepted.
Parameter | Type | Description |
---|---|---|
attributes
|
Array of Object | Array of attribute objects, each with the fields described for the result. |
match_type
|
String or Integer | [Required] The attribute name or type. |
If a matching attribute is found, the get_first_attribute
method returns an attribute
object with the following fields.
Field | Type | Description |
---|---|---|
.name
|
String |
The name of the RADIUS attribute, e.g. User-Name .This field will only be present if the attribute is supported in N2::RADIUS::Codec .
|
.type
|
Integer | [Required] The code that identifies the RADIUS attribute. |
.vendor_id
|
Integer |
The identifier of the vendor that defines the attribute if the attribute is a vendor-specific attribute. This field will only be present if the attribute is supported in N2::RADIUS::Codec .
|
.data_type
|
String |
[Required] One of enum , ifid , integer , ipv4addr , ipv4prefix ,
ipv6addr , ipv6prefix , string , other , text ,
time , unknown .
|
.value
|
Various |
[Required] The attribute's value. For most attributes this will be a SCALAR of the appropriate type. For attributes with data type other this may be a table.
|
.value_name
|
String |
The name associated with value .This field will only be present if the attribute .data_type is enum and the value mapping is defined in N2::RADIUS::Codec .
|
.get_first_attribute_value [Synchronous]
The get_first_attribute_value
method searches an array of attributes, returning the value of the first attribute with matching name or type. The following parameters are accepted.
Parameter | Type | Description |
---|---|---|
attributes
|
Array of Object |
Array of attribute objects, each with the fields described for the get_first_attribute result.
|
match_type
|
String or Integer | [Required] The attribute name or type. |
If a matching attribute is found, the get_first_attribute_value
method returns that attribute’s value.
For most attributes this will be a SCALAR of the appropriate type.
For attributes with data type other
this may be a table.
.get_all_attributes [Synchronous]
The get_all_attributes
method searches an array of attributes, returning all attributes with matching name or type. The following parameters are accepted.
Parameter | Type | Description |
---|---|---|
attributes
|
Array of Object |
Array of attribute objects, each with the fields described for the get_first_attribute result.
|
match_type
|
String or Integer | [Required] The attribute name or type. |
If at least one matching attribute is found, the get_all_attributes
method returns an array of attribute
objects as described for get_first_attribute
.
Otherwise an empty array is returned.
Built-In N2::RADIUS::Codec Packet Types
The following list of packet type names is built-in to the N2::RADIUS::Codec
encoding
and decoding library. Packet types not in this list may be referenced by numeric code.
Name | Code |
---|---|
Access-Request | 1 |
Access-Accept | 2 |
Access-Reject | 3 |
Accounting-Request | 4 |
Accounting-Response | 5 |
Access-Challenge | 11 |
Disconnect-Request | 40 |
Disconnect-ACK | 41 |
Disconnect-NAK | 42 |
CoA-Request | 43 |
CoA-ACK | 44 |
CoA-NAK | 45 |
Built-In N2::RADIUS::Codec Attributes
The following list of attributes is built-in to the N2::RADIUS::Codec
encoding and
decoding library.
Name | Type | Data Type |
---|---|---|
User-Name | 1 | string |
User-Password | 2 | string |
CHAP-Password | 3 | other |
NAS-IP-Address | 4 | ipv4addr |
NAS-Port | 5 | integer |
Service-Type | 6 | enum |
Framed-Protocol | 7 | enum |
Framed-IP-Address | 8 | ipv4addr |
Framed-IP-Netmask | 9 | ipv4addr |
Framed-Routing | 10 | enum |
Filter-Id | 11 | text |
Framed-MTU | 12 | integer |
Framed-Compression | 13 | enum |
Login-IP-Host | 14 | ipv4addr |
Login-Service | 15 | enum |
Login-TCP-Port | 16 | integer |
Reply-Message | 18 | text |
Callback-Number | 19 | string |
Callback-Id | 20 | string |
Framed-Route | 22 | text |
Framed-IPX-Network | 23 | integer |
State | 24 | string |
Class | 25 | string |
Vendor-Specific | 26 | other |
Session-Timeout | 27 | integer |
Idle-Timeout | 28 | integer |
Termination-Action | 29 | enum |
Called-Station-Id | 30 | string |
Calling-Station-Id | 31 | string |
NAS-Identifier | 32 | string |
Proxy-State | 33 | string |
Login-LAT-Service | 34 | string |
Login-LAT-Node | 35 | string |
Login-LAT-Group | 36 | string |
Framed-AppleTalk-Link | 37 | integer |
Framed-AppleTalk-Network | 38 | integer |
Framed-AppleTalk-Zone | 39 | string |
Acct-Status-Type | 40 | enum |
Acct-Delay-Time | 41 | integer |
Acct-Input-Octets | 42 | integer |
Acct-Output-Octets | 43 | integer |
Acct-Session-Id | 44 | text |
Acct-Authentic | 45 | enum |
Acct-Session-Time | 46 | integer |
Acct-Input-Packets | 47 | integer |
Acct-Output-Packets | 48 | integer |
Acct-Terminate-Cause | 49 | enum |
Acct-Multi-Session-Id | 50 | text |
Acct-Link-Count | 51 | integer |
Acct-Input-Gigawords | 52 | integer |
Acct-Output-Gigawords | 53 | integer |
Event-Timestamp | 55 | time |
Egress-VLANID | 56 | other |
Ingress-Filters | 57 | enum |
Egress-VLAN-Name | 58 | other |
User-Priority-Table | 59 | string |
CHAP-Challenge | 60 | string |
NAS-Port-Type | 61 | enum |
Port-Limit | 62 | integer |
Login-LAT-Port | 63 | string |
Tunnel-Type | 64 | other |
Tunnel-Medium-Type | 65 | other |
Tunnel-Client-Endpoint | 66 | other |
Tunnel-Server-Endpoint | 67 | other |
Tunnel-Password | 69 | other |
ARAP-Password | 70 | other |
ARAP-Features | 71 | other |
ARAP-Zone-Access | 72 | enum |
ARAP-Security | 73 | integer |
ARAP-Security-Data | 74 | string |
Password-Retry | 75 | integer |
Prompt | 76 | enum |
Connect-Info | 77 | text |
Configuration-Token | 78 | string |
EAP-Message | 79 | string |
Message-Authenticator | 80 | string |
Tunnel-Private-Group-ID | 81 | other |
Tunnel-Assignment-ID | 82 | other |
Tunnel-Preference | 83 | other |
ARAP-Challenge-Response | 84 | string |
Acct-Interim-Interval | 85 | integer |
NAS-Port-Id | 87 | text |
Framed-Pool | 88 | text |
Chargeable-User-Identity | 89 | string |
Tunnel-Client-Auth-ID | 90 | other |
Tunnel-Server-Auth-ID | 91 | other |
NAS-Filter-Rule | 92 | string |
Originating-Line-Info | 94 | string |
NAS-IPv6-Address | 95 | ipv6addr |
Framed-Interface-Id | 96 | ifid |
Framed-IPv6-Prefix | 97 | ipv6prefix |
Login-IPv6-Host | 98 | ipv6addr |
Framed-IPv6-Route | 99 | text |
Framed-IPv6-Pool | 100 | text |
Error-Cause | 101 | enum |
Delegated-IPv6-Prefix | 123 | ipv6prefix |
Vendor ID = 311 | ||
MS-CHAP-Response | 1 | other |
MS-CHAP-Error | 2 | other |
MS-CHAP-CPW-1 | 3 | other |
MS-CHAP-CPW-2 | 4 | other |
MS-CHAP-LM-Enc-PW | 5 | other |
MS-CHAP-NT-Enc-PW | 6 | other |
MS-MPPE-Encryption-Policy | 7 | enum |
MS-MPPE-Encryption-Types | 8 | integer |
MS-RAS-Vendor | 9 | integer |
MS-CHAP-Domain | 10 | other |
MS-CHAP-Challenge | 11 | string |
MS-CHAP-MPPE-Keys | 12 | string |
MS-BAP-Usage | 13 | enum |
MS-Link-Utilization-Threshold | 14 | integer |
MS-Link-Drop-Time-Limit | 15 | integer |
MS-MPPE-Send-Key | 16 | other |
MS-MPPE-Recv-Key | 17 | other |
MS-RAS-Version | 18 | string |
MS-Old-ARAP-Password | 19 | string |
MS-New-ARAP-Password | 20 | string |
MS-ARAP-Password-Change-Reason | 21 | enum |
MS-Filter | 22 | string |
MS-Acct-Auth-Type | 23 | enum |
MS-Acct-EAP-Type | 24 | enum |
MS-CHAP2-Response | 25 | other |
MS-CHAP2-Success | 26 | other |
MS-CHAP2-CPW | 27 | other |
MS-Primary-DNS-Server | 28 | ipv4addr |
MS-Secondary-DNS-Server | 29 | ipv4addr |
MS-Primary-NBNS-Server | 30 | ipv4addr |
MS-Secondary-NBNS-Server | 31 | ipv4addr |
MS-ARAP-Challenge | 33 | string |
Vendor ID = 10415 | ||
3GPP-IMSI | 1 | text |
3GPP-Charging-Id | 2 | integer |
3GPP-PDP-Type | 3 | enum |
3GPP-CG-Address | 4 | ipv4addr |
3GPP-GPRS-Negotiated-QoS-Profile | 5 | text |
3GPP-SGSN-Address | 6 | ipv4addr |
3GPP-GGSN-Address | 7 | ipv4addr |
3GPP-IMSI-MCC-MNC | 8 | text |
3GPP-GGSN-MCC-MNC | 9 | text |
3GPP-NSAPI | 10 | text |
3GPP-Session-Stop-Indicator | 11 | string |
3GPP-Selection-Mode | 12 | text |
3GPP-Charging-Characteristics | 13 | text |
3GPP-CG-IPv6-Address | 14 | ipv6addr |
3GPP-SGSN-IPv6-Address | 15 | ipv6addr |
3GPP-GGSN-IPv6-Address | 16 | ipv6addr |
3GPP-IPv6-DNS-Servers | 17 | other |
3GPP-SGSN-MCC-MNC | 18 | text |
3GPP-Teardown-Indicator | 19 | string |
3GPP-IMEISV | 20 | text |
3GPP-RAT-Type | 21 | string |
3GPP-User-Location-Info | 22 | other |
3GPP-MS-TimeZone | 23 | other |
3GPP-CAMEL-Charging-Info | 24 | string |
3GPP-Packet-Filter | 25 | other |
3GPP-Negotiated-DSCP | 26 | string |
3GPP-Allocate-IP-Type | 27 | string |
External-Identifier | 28 | text |
TWAN-Identifier | 29 | string |
3GPP-User-Location-Info-Time | 30 | integer |
3GPP-Secondary-RAT-Usage | 31 | other |
3GPP-UE-Local-IP-Address | 32 | other |
3GPP-UE-Source-Port | 33 | other |