FOX Lua Agent
Introduction
The FoxLuaAgent is an asynchronous helper for Lua scripts running within the LogicApp. It is used for sending out FOX client requests.
- The FOX Client Agent may be used by any Lua script, regardless of which Service initiated that script.
The FoxLuaAgent communicates with one or more instances of the FoxApp which can be used to communicate with one or more external FOX servers.
The FoxLuaAgent communicates with the FoxApp using the FOX-C-… messages.
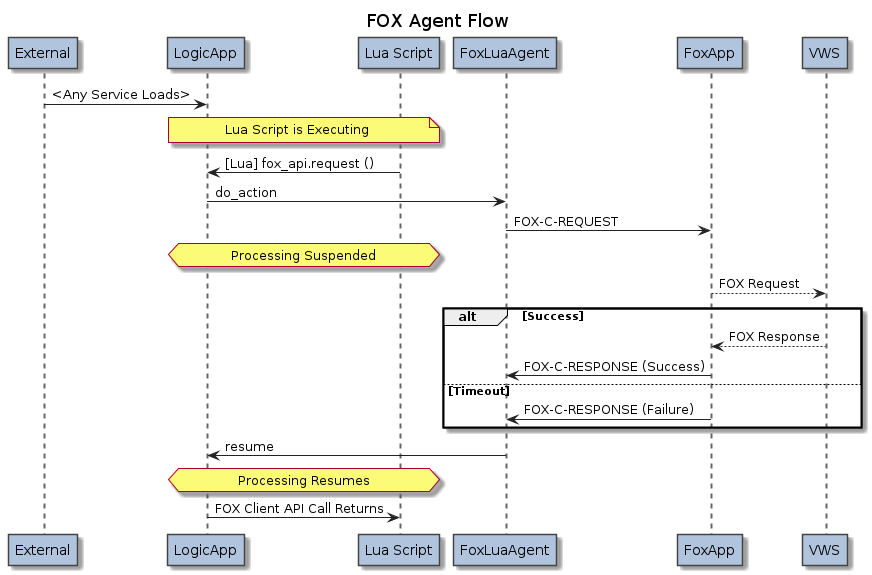
FOX Client Agent methods are accessed via the “n2.n2svcd.fox_agent” module:
local fox_api = require "n2.n2svcd.fox_agent"
Configuring FoxLuaAgent
The FoxLuaAgent is configured within a LogicApp.
<?xml version="1.0" encoding="utf-8"?>
<n2svcd>
...
<applications>
...
<application name="Logic" module="LogicApp">
<include>
<lib>../apps/logic/lib</lib>
</include>
<parameters>
...
<parameter name="default_fox_app_name" value="FOX-CLIENT"/>
</parameters>
<config>
<services>
...
</services>
<agents>
<agent module="FoxApp::FoxLuaAgent" libs="../apps/fox/lib"/>
</agents>
</config>
</application>
...
</application>
...
</n2svcd>
Under normal installation, following agent
attributes apply:
Parameter Name | Type | XML Type | Description |
---|---|---|---|
module
|
FoxApp::FoxLuaAgent
|
Attribute | [Required] The module name containing the Lua Agent code. |
libs
|
../apps/fox/lib
|
Attribute |
Location of the module for FoxLuaAgent.
|
In addition, the FoxLuaAgent must be configured with the name of the FoxApp with which
it will communicate. This is configured within the parameters
of the containing
LogicApp
.
Parameter Name | Type | XML Type | Description |
---|---|---|---|
parameters
|
Array | Element |
Array of name = value Parameters for this Application instance.
|
.default_fox_app_name
|
String | Attribute | Default name for the FoxApp with which FoxLuaAgent will communicate. |
.fox_app_name_
|
String | Attribute |
Use this format when FoxLuaAgent will communicate with more than one FoxApp .(Default = FoxLuaAgent uses only the default route/FOX endpoint). |
The FoxLuaAgent API
All methods may raise a Lua Error in the case of exception, including:
- Invalid input parameters supplied by Lua script.
- Unexpected results structure returned from FoxApp.
- Processing error occurred at FoxApp.
- Timeout occurred at FoxApp.
Note that if you are creating a new service, you may not necessarily need to code directly
against this API. Instead, you may want to consider using the ccs.lua
helper library.
This is a high-level service-implementation API for manipulating common NCC CCS subscriber
details.
.request [Asynchronous]
The request
method accepts an request
object parameter with the following attributes.
Field | Type | Description |
---|---|---|
be_id
|
String |
The billing engine ID for the subscriber configured in CCS_DOMAIN .DOMAIN_ID .You will normally read this from the CCS_ACCT .BE_ACCT_ENGINE_ID database table.If only one VWS pair is present, this may be hard-coded. |
request
|
Object |
Description of the FOX request structure. You must specify TYPE . You may specify BODY . The HEAD will have defaults set.You must specify HEAD .CMID if you wish to make a follow-up request within a session.This structure is specified by Oracle. |
The request
method returns a FOX response
object with the following attributes.
Parameter | Type | Description |
---|---|---|
TYPE
|
ACK /NACK /EXCP
|
The response type. These values are specified by Oracle. |
HEAD
|
Object |
Container for header fields returned in the response. This structure is specified by Oracle. |
BODY
|
Object |
Container for body fields returned in the response. This structure is specified by Oracle. |
Example (Wallet Info FOX Request):
local n2svcd = require "n2.n2svcd"
local fox_api = require "n2.n2svcd.fox_agent"
local soap_args = ...
local be_id = 1
local request = {
TYPE = 'WI',
BODY = {
AREF = aref,
WALT = walt,
WTYP = wtyp
}
}
local response = fox_api.request (be_id, request)
if (response.ACTN ~= 'ACK') then
error ("VWS Wallet Info Failed: [" .. (response.BODY.CODE or "?")
.. "-" .. (response.BODY.WHAT or "Unknown Error") .. "]")
end
for bals_k, bals_v in pairs (response.BODY.BALS) do
...
end
return
[Fragment] Example (Wallet Delete FOX request):
local be_id = 1
local request = {
TYPE = 'WD',
BODY = {
ACTY = acty,
CLI = cli,
WALT = walt,
WTYP = wtyp
}
}
local response = fox_api.request (be_id, request)
if (response.ACTN ~= 'ACK') then
error ("VWS Wallet Delete Failed: [" .. (response.BODY.CODE or "?")
.. "-" .. (response.BODY.WHAT or "Unknown Error") .. "]")
end
Supported NCC::FOX Codec Fields
The Oracle FOX protocol design does not support dynamically or user-defined field types. All field names must be pre-defined. This means that the FOX codec used by the LUA agent has a static pre-defined list of field names and associated types.
The BODY
fields currently coded into the FOX codec are:
Field Structure | FOX Type |
---|---|
.BODY | MAP |
.BODY.ABAL | ARRAY |
.BODY.ABAL.* | MAP |
.BODY.ABAL.*.BKTS | ARRAY |
.BODY.ABAL.*.BKTS.* | MAP |
.BODY.ABAL.*.BKTS.*.AASS | STRING |
.BODY.ABAL.*.BKTS.*.EXPR | DATE |
.BODY.ABAL.*.BKTS.*.ID | INTEGER |
.BODY.ABAL.*.BKTS.*.LUSE | DATE |
.BODY.ABAL.*.BKTS.*.STDT | DATE |
.BODY.ABAL.*.BKTS.*.SUBN | STRING |
.BODY.ABAL.*.BKTS.*.UVAL | INTEGER |
.BODY.ABAL.*.BKTS.*.VAL | INTEGER |
.BODY.ABAL.*.BTYP | INTEGER |
.BODY.ABAL.*.BUNT | INTEGER |
.BODY.ABAL.*.LIMT | SYMBOL |
.BODY.ABAL.*.STOT | INTEGER |
.BODY.ABAL.*.UTOT | INTEGER |
.BODY.ACTV | DATE |
.BODY.ACTY | INTEGER |
.BODY.APOL | SYMBOL |
.BODY.AREF | INTEGER |
.BODY.BALS | ARRAY |
.BODY.BALS.* | MAP |
.BODY.BALS.*.BKTS | ARRAY |
.BODY.BALS.*.BKTS.* | MAP |
.BODY.BALS.*.BKTS.*.EXPR | DATE |
.BODY.BALS.*.BKTS.*.ID | INTEGER |
.BODY.BALS.*.BKTS.*.LUSE | DATE |
.BODY.BALS.*.BKTS.*.STDT | DATE |
.BODY.BALS.*.BKTS.*.SUBN | STRING |
.BODY.BALS.*.BKTS.*.UVAL | INTEGER |
.BODY.BALS.*.BKTS.*.VAL | INTEGER |
.BODY.BALS.*.BTYP | INTEGER |
.BODY.BALS.*.BUNT | INTEGER |
.BODY.BALS.*.LIMT | SYMBOL |
.BODY.BALS.*.STOT | INTEGER |
.BODY.BALS.*.UTOT | INTEGER |
.BODY.BPOL | SYMBOL |
.BODY.CDAT | DATE |
.BODY.CDR | ARRAY |
.BODY.CDR.* | MAP |
.BODY.CDR.*.TAG | STRING |
.BODY.CDR.*.VAL | STRING |
.BODY.CLI | STRING |
.BODY.CODE | STRING |
.BODY.DN | STRING |
.BODY.EXPR | DATE |
.BODY.LUSE | DATE |
.BODY.MAXC | INTEGER |
.BODY.NAME | STRING |
.BODY.NUM | INTEGER |
.BODY.RBAA | ARRAY |
.BODY.RBAA.* | MAP |
.BODY.RBAA.*.BTYP | INTEGER |
.BODY.RBAA.*.RBIA | ARRAY |
.BODY.RBAA.*.RBIA.* | MAP |
.BODY.RBAA.*.RBIA.*.BKID | INTEGER |
.BODY.RBAA.*.RBIA.*.BPOL | SYMBOL |
.BODY.RBAA.*.RBIA.*.VAL | INTEGER |
.BODY.RESN | STRING |
.BODY.RWI | NULL |
.BODY.SATI | INTEGER |
.BODY.SCID | STRING |
.BODY.SCLI | STRING |
.BODY.SCUR | INTEGER |
.BODY.SDID | INTEGER |
.BODY.SDNF | NULL |
.BODY.SPID | INTEGER |
.BODY.SSUB | INTEGER |
.BODY.STAT | SYMBOL |
.BODY.STRV | STRING |
.BODY.SWID | INTEGER |
.BODY.SWTI | INTEGER |
.BODY.TATI | INTEGER |
.BODY.TDID | INTEGER |
.BODY.TSUB | INTEGER |
.BODY.TWID | INTEGER |
.BODY.TZ | STRING |
.BODY.UCUR | INTEGER |
.BODY.WALT | INTEGER |
.BODY.WHAT | STRING |
.BODY.WTYP | INTEGER |